Quick Dashboarding With Panel
Published October 27, 2020
Dillon Roach
A bespoke, polished data science dashboard can be a beautiful thing for anyone looking to make data-driven decisions. And yet, not every project can afford setting up elaborate dashboards that cost money and developer time.
In this post, we show you how to construct a quick dashboard using Panel & Python without ever leaving the comfort of your Jupyter notebook. Before you start, download the demonstration video.
For this example, we want to create a dynamic plot dashboard displaying historical trends of popular (user-supplied) baby names.
While the historical trends of name registrations are not at the forefront of business decisions, similar name-indexed data queries could involve stock indexes, product names in your catalog (or a competitor's), or perhaps predictions of future trends for your named-apparel business.
This baby-name data originally comes from the US Social Security open data; we have modified it for ease of use and to off-load some pre-processing needed for the final plots.
For each observation, we have preserved Year, Name, and Gender as features from the original data. We also have a feature Normalized that represents the percentage of all names within a given year.
We could add names common to M & F genders—say Dillon (M) & Dillon (F)—but we leave them distinct here to preserve their individual trends.
We present a random slice of the data below to build intuition.
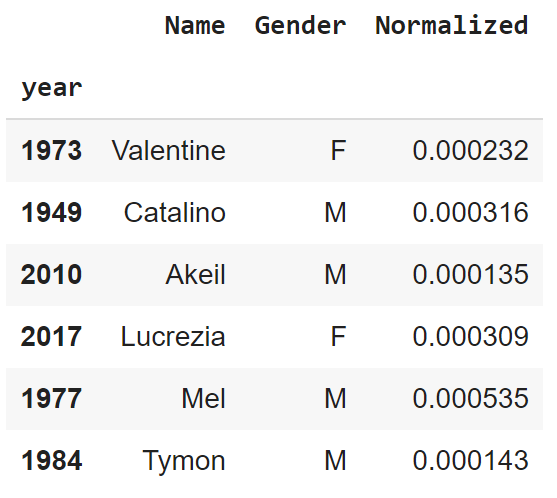
With our data in hand, we first want to define a plotter
function. This
function produces plots of historical trends of registered baby names over a
range of years with certain scalings (all user-specified). If you're unfamiliar
with Python, it's enough to simply say that this function produces the plot we
want without any interactive components. Without Panel, you can invoke this
function yourself to generate plots in Jupyter using different input values
supplied by hand.
If calling plotter
itself were enough, we'd be done. In this case, we want to
share a plotting tool with a boss or friends. This means we want some
interactive elements for our dashboard: widgets.
Widgets are often the basic building blocks of a Panel dashboard. These
components are familiar to anyone who has seen web forms previously. For the
use-case here, we need only three types of Panel widget: TextInput
(to enter
names), a RangeSlider
(to select the years to plot), and Checkbox
(to toggle
optional metrics).
The function panel.interact
makes it straightforward to connect the plotter
function with the preceding widgets. We simply supply the function (plotter
)
as the first input argument to panel.interact
. The remaining input arguments
associate widgets with plotter
's input arguments. Be certain that the widget
output data types match with plotter
's requirements. For example, the input
years
should be a (sorted) list or tuple of two distinct integers; a
RangeSlider
with specified values for start & stop provides a valid tuple.
Panel also enables arranging elements of your dashboard with Row
and Column
objects. For instance, below, dashboard[0]
contains all the input widgets,
while dashboard[1]
is the output of the plotter.
The addition of .servable()
to your panel layout allows the entire notebook to be called by
from the command line (provided you have Panel installed). This allows multiple clients to connect to this service via web browsers.
There you have it—a quick and easy option for dashbording without breaking a sweat. That's not all that Panel can do, but for a simple framework for getting a UI wrapped around your functions, it's hard to beat.
Here is a link to the Binder for running this notebook.
Here is the data for this exercise.